- Why did I use Django and hosted it on Digital Ocean?
- Why did I migrate?
- First attempt: Adopt a mixed-cloud strategy of Digital Ocean + AWS
- A new dilemna: Single page or multi page application?
- Universal application: the middle ground of single and multi page application
- Before and after
- The migration process
- What I'd miss about my old stack
Why did I use Django and hosted it on Digital Ocean?
In 2021, I left my web development job and decided to take a Masters in Data Science.
Before the course, I took some online courses about data science.
I had this idea of building a website where I demonstrate my proficiency in both and how to combine them.
At that time, I only knew about Digital Ocean and it was the platform used by my old job.
It was a decent tech stack, as I enjoyed the following benefits.
Django comes in a package, including security and a database
Firstly, Python must be installed in your system and it's best to set up a virtual environment, either with pipenv or venv. Make sure the environment is activated.
With django installed, use the following command:django-admin startproject sitename
I prefer moving all the contents in the sitename file one level higher.
Django allows users to split their django project into smaller components, known as apps. Each app comes with its own administrative settings and urls. More on this in the next section.python manage.py startapp appname
Notice how django already came with security and middleware in settings.py. Absolutely Fantastic!
Use the following three commands when there are new files added and to start the test server.python manage.py makemigrations
python manage.py migrate
python manage.py runserver
Notice how a db.sqlite3 file is generated the first time you run these three commands. That's a basic database created for you!
When you visit http://127.0.0.1:8000/admin/, you are able to access the database. It's that convenient.
Thus, it makes it very easy for someone with some python experience to get started with their own website. In my case, because python is also used in data science, I can put data science logics in my django, usually in views.py.Django splits website into smaller app components
As I was explaining earlier, each app has its own urls. In my old website, I had 3 app components, home, scenarios and legacy in their respective views.py. Each app has its own url and in the url.py at the root directory, I can register all of them.
Django will nicely add to your url, based on the app name. For example I have a spellchecker_1 in my scenarios app, so the url would be http://127.0.0.1:8000/scenarios/spellchecker_1/
So, this feature makes website navigation very systematic.Deploying Django to Digital Ocean is easy and affordable
Django has this app platform that charges only like 5 dollars per month and they provide a super comprehensive deployment guide . Their tutorial even teaches how to link your git repository to the deployment. In other words, you can make changes to your django repository, push to github and Digital Ocean will deploy the pushed codes. Fun fact, you can totally skip the step where they tell you to use postgres, if you are not using any database (I did not). One time, I was building a super basic e-commerce website for my sister and we just stick with the default sqlite generated by django. Was it best practice to do so? No. But, we were'nt charge any money for database.
Why did I migrate?
Yes, Django and Digital Ocean combination was really convenient and affordable.
It was like living in a small and cozy house in a winter, with the food all stocked up and without even needing to leave the couch.
I used this tech stack for a year, until after taking my AWS Certified Cloud Practitioner exam and how its important
to avoid monolithic systems and instead, decouple with API Gateway and lambda.
I then realize the problems with my tech stack.
Django libraries and data science libraries were all installed in the same system
Because I tend to allow users to submit form and let the data science prediction logic work in my views.py, it leads to quite a huge dependencies list. It is very convenient to do so, but if I were to add a new Python library to my system for every project, more and more storage space will be used up to install them. Plus, different projects may use different versions of the same library.
Static files are stored TWICE!
According to Django ,python manage.py collectstatic
is a command for collecting all the static files (images, audio etc) in all Django apps, into a single location for serving them in production. And of course, it basically doubles my storage space needed for static files!
For example, I have two of the exact same base.css files. Not only is this taking more space, but it leads to confusion during editing, I cannot edit the duplicated one meant for production, I'm supposed to edit the original one and do apython manage.py collectstatic
to update the static file for production.
Yes, I could add a .gitignore to not push the non-production staticfiles, but you get my point.Redeploying is painful and slow
When you put your frontend and backend all in a single project and deployment, even a slight typo in html requires you to redeploy everything! Not only that, it is very hard to debug, because it's all one big monolith! Say, a form doesn't work, is it a frontend or backend issue? It could be neither, as I was once asked to deploy all the way to the U.S, instead of Singapore, due to unknown issue from the cloud provider. I kept failing to deploy and each trial took me more than an hour, before I finally was told of the actual issue.
First attempt: Adopt a mixed-cloud strategy of Digital Ocean + AWS
I put my production static files into an S3 bucket, as suggested by this tutorial . Then, I keep only the Python web development libraries, by putting the data science functions into lambda and API Gateway.
A new dilemna: Single page or multi page application?
I was using both VueJS and Django in my pages.
As I was explaining earlier, Django is secure and easy to set up, but I did not explain why I used Vue.
VueJS, as explained on their official website
, has two core features, which are declarative rendering and reactivity.
Both Django and VueJS allow the users to use templates, for example,
I can break a HTML page into components, such as page footer, and reuse them in other pages.
However, Django does not have the reactivity.
Reactivity allows HTML components to change according to JavaScript.
For example, in the JavaScript, the variable, height, changed from 150 to 170.
If you have linked this variable to a HTML component, say a bar chart,
then the bar chart gets updated instantly, without the need to write a function
in JavaScript to update the html component.
While it was possible to use both VueJS and Django at the same time,
the integration was kinda awkward, because they both use curly brackets in html components.
As you can see, I use curly brackets for Django, to get the location of static files like images. I have declared my delimiters as square brackets for VueJS to use, so that there is no conflict for curly brackets.
But, I still miss out VueJS features such as routing. With routing, when user navigates between webpages in the same website, there was no need to reload the entire page!
It is not really a huge issue, as many websites still use this, but as a former web application developer, it just bothers me. Also, in Django, when forms are submitted, entire page is reloaded too. Back then, in the web application I built for my work, APIs are called without reloading the page.
Some Googling around showed that this is related to the debate betweeen whether to use single page application (SPA) or multi page application (MPA).
SPAs are modern websites that act like mobile applications. With just a single page, user can perform a lot of actions, and not just limited to reading texts. For example, in my old job, the SPA could be used to drag and drop nodes and link them. Human resource managers could assign tasks to employees in each node. This all occured in the browser, within the same page!
MPAs are more like traditional websites. While each navigation requires reloading the entire page, it does come with the huge advantage of being more SEO friendly.
I was really stuck between these two options. I know I will be writing about my projects, but I also want to allow users to test out my projects easily and quickly, and make the website reflect my work experience as someone who has used modern web frameworks for work.
Universal application: the middle ground of single and multi page application
Luckily for me, I came across a VueJS library known as NuxtJS.
NuxtJS is a library for building universal application.
A universal application has the features of SPAs, while retaining the SEO friendliness of MPAs.
This is made possible through server-side rendering (SSR).
In conventional VueJS SPA, the search engine crawlers tend to struggle to find things to crawl.
In SSR, there are already HTML content pre-rendered for the search engine crawlers.
So yes, this website, as you can see, is built using NuxtJS!
Before and after
Let me show you a comprison between my old stack (Django + Vue) vs. NuxtJS.
The following videos and images show the differences side by side, with NuxtJS on the left, Django on the right.
In terms of navigation, although the speed is roughly equal, there is no need for any reloading in NuxtJS. In fact, there's an animation in Nuxt.JS that gets played during page navigation.
When calling the backend (making prediction in python), using API in NuxtJS does not result in page refreshing.
Without cloudfront, Django has higher metrics.
The gap is reduced slightly with cloudfront.
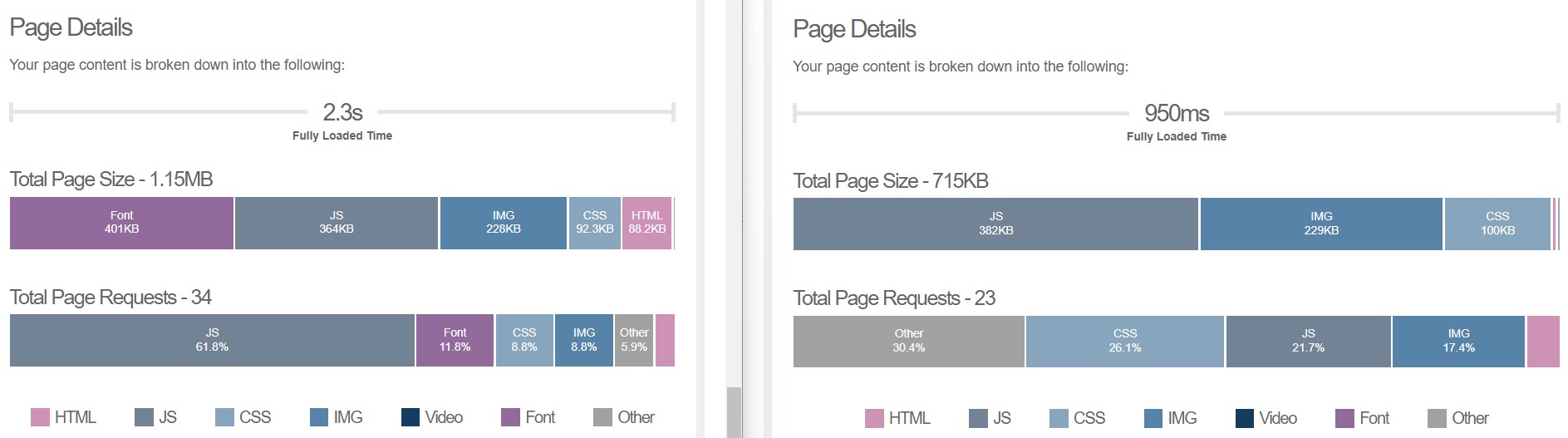
This may be due to all the fonts that come with NuxtJS, specifically, since I used Vuetify, leading to more stuff to be loaded. In places like my project directory page, I added icons for each project, as opposed to how originally, they were just a list of hyperlinks.
Nevertheless, cloudfront still reduced the loading time of the files.
The most important question is not about the metrics, but rather, is the increased files worth it? I would say yes, because the UI becomes a lot more modernized and this is not something that is measurable.
The migration process
Rebuilding the website in Nuxt.JS
It's my first time using it, but NuxtJS feels very intuitive. Maybe because I had work experience in VueJS.
I just need to create pages by creating .vue files in the pages directory. The default.vue was generated by Nuxt too, you can set the background, page header etc and it will be displayed in all pages. Then, there's the components folder, where I have html components that I can reuse, like my page footer, where I put my contact details.Transitioning from .html to .vue files
In my Django, the web pages were stored as html files. For the most part, it wasn't an issue to transfer them to .vue file. However, in some cases, VueJS does not allow certain JavaScript files to be used in the script tag. I just used iframes for such pages. These pages include Pandemic Monument, a page with Tableau integration and the grammar simulations I built with basic HTML and SVG from a long time ago, now saved in my project directory page.Deploying to AWS Amplify
I just followed every step in this tutorial. It worked perfectly. These days, all I need to do is to useamply publish
What I'd miss about my old stack
It is true that AWS has the biggest market share, but I found out that
AWS PaaS services such as Beanstalk does not support latest Django.
If I were to deploy another Django, I would still choose Digital Ocean.
When I tried to deploy my Django stack, which was using the latest version,
it did not work and I was told that Beanstalk does not support latest Django version.
Personally, I like the support given from Digital Ocean team too!
On top of that, it does not involve such a steep learning curve, compared to AWS.
If I hadn't taken an AWS Udemy course and its exam, I would still stick with Digital Ocean.